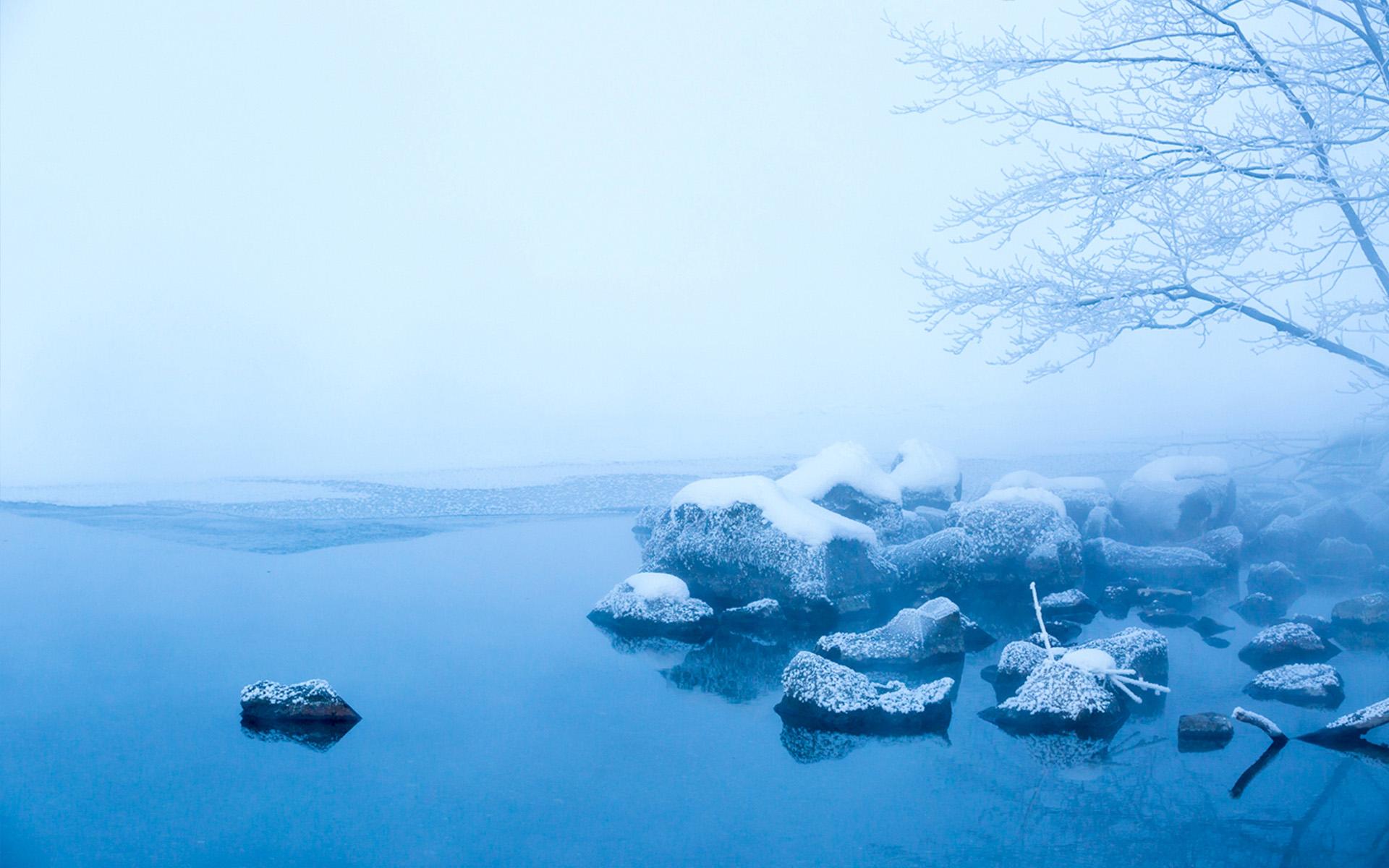
vue3 + TS + Vite + Vuex4 + Element-Plus 创建项目
前端小菜-贺俊兰 2021-03-29 VUE
# 创建项目
输入命令按步骤往下即可,详细 API 可参考 Vite 官方文档
npm init @vitejs/app
yarn create @vitejs/app
选择 Vue - TS 版本
1
2
3
4
5
2
3
4
5
# 引入 Element-Plus
npm i element-plus
yarn add element-plus
1
2
3
2
3
# 引入 Vuex4
npm install vuex@next
yarn add vuex@next
1
2
3
2
3
# 配置修改
main.ts
import { createApp } from "vue";
import App from "./App.vue";
import { store } from "./store";
import "element-plus/lib/theme-chalk/index.css";
import ElementPlus from "element-plus";
const app = createApp(App);
app.use(ElementPlus);
app.use(store);
app.mount("#app");
1
2
3
4
5
6
7
8
9
10
2
3
4
5
6
7
8
9
10
# Vuex 模块化
新建文件
--- store
--- modules 模块化文件
--- test.ts
--- index.ts
1
2
3
4
2
3
4
test.ts
export default {
namespaced: true,
state: {
count: 10,
},
mutations: {
SET_COUNT(state: any) {
state.count++;
},
},
actions: {
set_count(ctx: any) {
ctx.commit("SET_COUNT");
},
},
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
index.ts
import { createStore } from "vuex";
import textModules from "./modules/test";
export const store = createStore({
modules: {
textModules,
},
});
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
# 测试
修改 HelloWorld
<template>
<h1>{{ msg }}</h1>
<h1>count: {{ count }}</h1>
<el-button type="success" @click="addCount">+1</el-button>
</template>
<script lang="ts">
import { defineComponent, computed } from "vue";
import { useStore } from "vuex";
export default defineComponent({
name: "HelloWorld",
props: {
msg: {
type: String,
required: true,
},
},
setup: () => {
const store = useStore();
const count = computed(() => {
return store.state.textModules.count;
});
return {
count,
addCount: () => store.dispatch("textModules/set_count"),
};
},
});
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
当出现 element 的按钮并能成功 count++,说明配置 ok